C++new和delete的使用
<上一节
下一节>
基本数据类型的动态分配
new和delete已经完全包含malloc和free的功能,并且更强大、方便、安全。使用动态分配内存时不能忘记释放内存,不要忘记出错处理!下面先看new和delete的基本使用方法。#include <iostream> using namespace std; int main ( ) { //基本数据类型 int *i = new int; //没有初始值 int *j = new int(100); //初始值为100 float *f = new float(3.1415f); //初始值为3.14159 cout << " i = " << *i << endl; cout << " j = " << *j << endl; cout << " f = " << *f << endl; //数组 int *iArr = new int[3]; for (int i=0; i<3; i++) { iArr[i] = (i+1)*10; cout << i << ": " << iArr[i] << endl; } //释放内存 delete i; delete j; delete f; delete []iArr; //注意数组的删除方法 return 0; }输出结果:
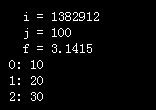
内存分配时的出错处理
一般有2种处理方法,一是根据指针是否为空来判断,一是用例外出错处理所抛出的“bad_alloc”来处理。#include <iostream> #include <new> using namespace std; int main ( ) { //判断指针是否为NULL double *arr = new double[100000]; if (!arr) { cout << "内存分配出错!" << endl; return 1; } delete []arr; //例外出错处理 try { double *p = new double[100000]; delete []p; } catch (bad_alloc xa) { cout << "内存分配出错!" << endl; return 1; } //强制例外时不抛出错误,这时必须要判断指针 double *ptr = new(nothrow) double[100000]; if (!ptr) { cout << "内存分配出错!" << endl; return 1; } delete []ptr; cout << "内存分配成功!" << endl; return 0; }输出结果:
内存分配成功!
用new产生类的实例
前面已经用了很多了。#include <iostream> using namespace std; class classA { int x; public: classA(int x) { this->x = x; } int getX() { return x; } }; int main ( ) { classA *p = new classA(200); //调用构造函数 if (!p) { cout << "内存分配出错!" << endl; return 1; } cout << "x = " << p->getX() << endl; delete p; //调用析构函数 return 0; }运行结果:
x = 200
<上一节
下一节>