Maven导入本地jar包
我们知道,Maven 是通过仓库对依赖进行管理的,当 Maven 项目需要某个依赖时,只要其 POM 中声明了依赖的坐标信息,Maven 就会自动从仓库中去下载该构件使用。但在实际的开发过程中,经常会遇到一种情况:某一个项目需要依赖于存储在本地的某个 jar 包,该 jar 包无法从任何仓库中下载的,这种依赖被称为外部依赖或本地依赖。那么这种依赖是如何声明的呢?
下面我们通过一个实例实例来介绍如何导入本地 jar 包。
1. 打开命令行窗口,跳转到 D:\maven 目录下,执行以下 mvn 命令,创建一个名为 secondMaven 的项目。
2. 更新 secondMaven 中 App 类的代码如下。
由以上代码可以看出,secondMaven 中的 App 类需要使用 helloMaven 中独有的 Util 类,即 secondMaven 需要依赖于 helloMaven。
3. 跳转到 helloMaven 所在的目录,执行以下 mvn 命令, 将该项目打包成 jar 文件。
命令执行执行结果如下。
4. 命令执行完成后,进入 D:\maven\helloMaven\target 目录,可以看到 Maven 已经将 helloMaven 项目打包成 helloMaven-1.0-SNAPSHOT.jar。
5. 修改 secondMaven 中 pom.xml 的配置如下。
在以上配置中,除了依赖的坐标信息外,外部依赖还使用了 scope 和 systemPath 两个元素。
6. 打开命令行窗口,跳转到 secondMaven 所在目录,执行以下 mvn 命令,进行编译。
命令执行结果如下。
7. 编译完成后,执行以下命令,设置临时环境变量。
8. 执行以下 Java 命令。
9. 结果如下图。
下面我们通过一个实例实例来介绍如何导入本地 jar 包。
1. 打开命令行窗口,跳转到 D:\maven 目录下,执行以下 mvn 命令,创建一个名为 secondMaven 的项目。
mvn archetype:generate -DgroupId=net.biancheng.www -DartifactId=secondMaven -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
2. 更新 secondMaven 中 App 类的代码如下。
package net.biancheng.www; import net.biancheng.www.Util;; public class App { public static void main(String[] args) { Util.printMessage("secondMaven"); } }
由以上代码可以看出,secondMaven 中的 App 类需要使用 helloMaven 中独有的 Util 类,即 secondMaven 需要依赖于 helloMaven。
3. 跳转到 helloMaven 所在的目录,执行以下 mvn 命令, 将该项目打包成 jar 文件。
mvn clean package
命令执行执行结果如下。
[INFO] Scanning for projects... [INFO] [INFO] --------------------< net.biancheng.www:helloMaven >-------------------- [INFO] Building helloMaven 1.0-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-clean-plugin:2.5:clean (default-clean) @ helloMaven --- [INFO] Deleting d:\maven\helloMaven\target [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ helloMaven --- [WARNING] Using platform encoding (GBK actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory d:\maven\helloMaven\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ helloMaven --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding GBK, i.e. build is platform dependent! [INFO] Compiling 2 source files to d:\maven\helloMaven\target\classes [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ helloMaven --- [WARNING] Using platform encoding (GBK actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory d:\maven\helloMaven\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.1:testCompile (default-testCompile) @ helloMaven --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding GBK, i.e. build is platform dependent! [INFO] Compiling 1 source file to d:\maven\helloMaven\target\test-classes [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ helloMaven --- [INFO] Surefire report directory: d:\maven\helloMaven\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running net.biancheng.www.AppTest Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.016 sec Results : Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] --- maven-jar-plugin:2.4:jar (default-jar) @ helloMaven --- [INFO] Building jar: d:\maven\helloMaven\target\helloMaven-1.0-SNAPSHOT.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 1.940 s [INFO] Finished at: 2021-03-03T13:27:34+08:00 [INFO] ------------------------------------------------------------------------
4. 命令执行完成后,进入 D:\maven\helloMaven\target 目录,可以看到 Maven 已经将 helloMaven 项目打包成 helloMaven-1.0-SNAPSHOT.jar。
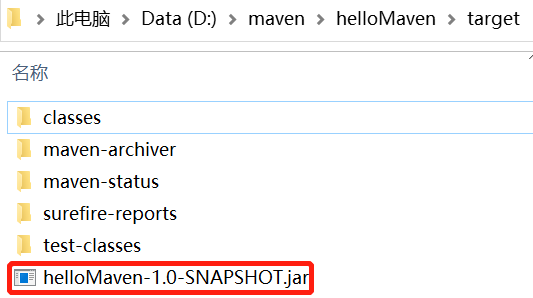
图1: helloMaven 打包结果
5. 修改 secondMaven 中 pom.xml 的配置如下。
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>net.biancheng.www</groupId> <artifactId>secondMaven</artifactId> <packaging>jar</packaging> <version>1.0-SNAPSHOT</version> <name>secondMaven</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <!--外部依赖--> <dependency> <groupId>net.biancheng.www</groupId> <artifactId>helloMaven</artifactId> <!--依赖范围--> <scope>system</scope> <version>1.0-SNAPSHOT</version> <!--依赖所在位置--> <systemPath>D:\maven\helloMaven\target\helloMaven-1.0-SNAPSHOT.jar</systemPath> </dependency> </dependencies> </project>
在以上配置中,除了依赖的坐标信息外,外部依赖还使用了 scope 和 systemPath 两个元素。
- scope 表示依赖范围,这里取值必须是 system,即系统。
- systemPath 表示依赖的本地构件的位置。
6. 打开命令行窗口,跳转到 secondMaven 所在目录,执行以下 mvn 命令,进行编译。
mvn clean compile
命令执行结果如下。
[WARNING] [WARNING] Some problems were encountered while building the effective model for net.biancheng.www:secondMaven:jar:1.0-SNAPSHOT [WARNING] 'dependencies.dependency.systemPath' for net.biancheng.www:helloMaven:jar should use a variable instead of a hard-coded path D:\maven\helloMaven\target\helloMaven-1.0-SNAPSHOT.jar @ line 23, column 16 [WARNING] [WARNING] It is highly recommended to fix these problems because they threaten the stability of your build. [WARNING] [WARNING] For this reason, future Maven versions might no longer support building such malformed projects. [WARNING] [INFO] [INFO] -------------------< net.biancheng.www:secondMaven >-------------------- [INFO] Building secondMaven 1.0-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-clean-plugin:2.5:clean (default-clean) @ secondMaven --- [INFO] Deleting d:\maven\secondMaven\target [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ secondMaven --- [WARNING] Using platform encoding (GBK actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory d:\maven\secondMaven\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ secondMaven --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding GBK, i.e. build is platform dependent! [INFO] Compiling 1 source file to d:\maven\secondMaven\target\classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 1.030 s [INFO] Finished at: 2021-03-03T14:05:00+08:00 [INFO] ------------------------------------------------------------------------
7. 编译完成后,执行以下命令,设置临时环境变量。
set classpath=%classpath%;D:\maven\helloMaven\target\helloMaven-1.0-SNAPSHOT.jar
8. 执行以下 Java 命令。
java net.biancheng.www.App
9. 结果如下图。
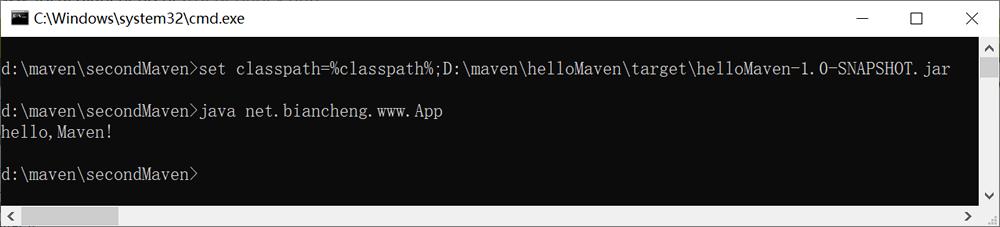
图2:执行结果