Go语言接口嵌套(小白必读)
接口嵌套是在一个接口中嵌套另一个接口,通过接口嵌套能使接口之间形成简单的继承关系,但接口之间不具备方法重写功能,即多个接口嵌套组成一个新的接口,每个接口的方法都是唯一的。其实现语法如下:
接口嵌套允许在不同接口中定义相同的方法名,但方法的参数和返回值必须一致,比如接口 leg 定义了方法 run(),接口 actions 允许定义同名方法 run(),但程序会将两个同名方法默认为同一个方法,所以同名方法的参数和返回值必须一致,否则程序执行时提示错误,如下图所示。
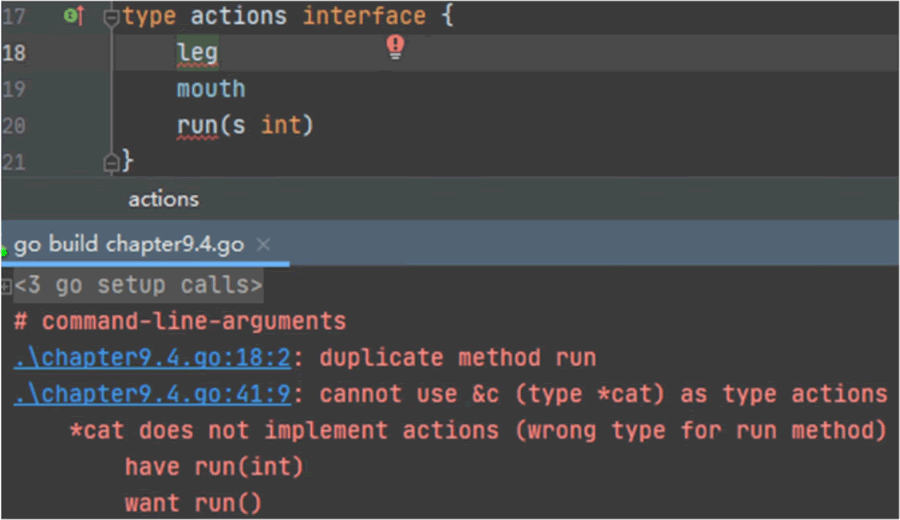
图 1 运行结果
接口嵌套通过多个接口组成一个新的接口,使代码设计变得更加灵活,为了降低接口之间的方法命名冲突,各个接口的方法名称尽量保持不同,建议使用接口名称+方法名称的组合方式命名,示例如下:
// 定义接口 type leg interface { run() } type mouth interface { speak(content string) } // 接口嵌套 type actions interface { leg mouth run() }上述语法定义了 3 个接口:leg、mouth 和 actions。接口 leg 和 mouth 分别定义了一个方法;接口 actions 嵌套了接口 leg 和 mouth,并定义了方法 run(),该方法与接口 leg 的方法 run() 同名。
接口嵌套允许在不同接口中定义相同的方法名,但方法的参数和返回值必须一致,比如接口 leg 定义了方法 run(),接口 actions 允许定义同名方法 run(),但程序会将两个同名方法默认为同一个方法,所以同名方法的参数和返回值必须一致,否则程序执行时提示错误,如下图所示。
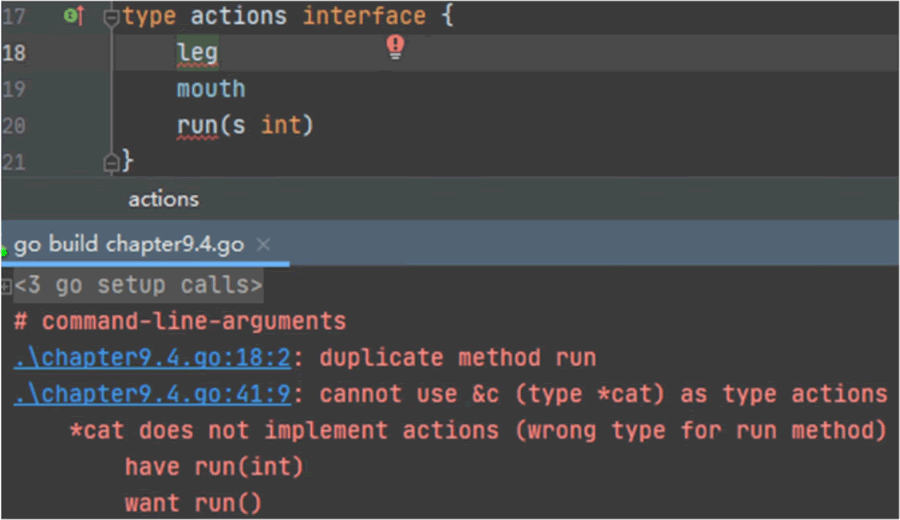
图 1 运行结果
接口嵌套通过多个接口组成一个新的接口,使代码设计变得更加灵活,为了降低接口之间的方法命名冲突,各个接口的方法名称尽量保持不同,建议使用接口名称+方法名称的组合方式命名,示例如下:
// 定义接口 type leg interface { leg_run() } type mouth interface { mouth_speak(content string) } // 接口嵌套 type actions interface { leg mouth actions_run() }通过接口名称+方法名称组合的方式定义各个接口方法,能直观掌握每个方法来自哪一个接口,这样能提高代码的可读性。根据上述语法,我们通过示例讲述如何使用接口嵌套,代码如下:
package main import ( "fmt" ) // 定义接口 type leg interface { leg_run() } type mouth interface { mouth_speak(content string) } // 接口嵌套 type actions interface { leg mouth actions_run() } // 定义结构体 type cat struct { name string } // 定义结构体方法 func (c *cat) mouth_speak(content string) { fmt.Printf("%v在说话:%v\n", c.name, content) } func (c *cat) leg_run() { fmt.Printf("%v在跑步\n", c.name) } func (c *cat) actions_run() { fmt.Printf("%v在奔跑\n", c.name) } // 定义函数 func factory() actions { c := cat{name: "凯蒂猫"} return &c } func main() { // 实例化结构体 c := factory() // 调用函数 c.leg_run() c.mouth_speak("喵喵喵喵") c.actions_run() }上述示例的实现过程如下:
- 定义接口 leg、mouth 和 actions,以及结构体 cat 和结构体方法 mouth_speak()、leg_run()、actions_run(),结构体方法分别对应接口 actions 的所有方法。
- 工厂函数 factory() 将结构体 cat 实例化,并且与接口 actions 进行绑定操作,生成接口变量 c 作为函数返回值。
- 主函数 main() 从工厂函数 factory() 获取接口变量 c,再由接口变量 c 分别调用方法 mouth_speak()、leg_run() 和 actions_run()。
凯蒂猫在跑步
凯蒂猫在说话:喵喵喵喵
凯蒂猫在奔跑