Python dict字典的用法(非常详细)
Python 中的字典(Dictionary)是一种无序的、可变的序列,字典元素以键值对的形式进行存储。列表和元组都是有序的序列,它们的元素在底层是顺序存放的。
字典是 Python 中唯一的一种映射类型,即元素之间是相互对应的。我们习惯将元素的索引称为键(key),键对应的元素称为值(value),键及其关联的值称为键值对。键值对示意图如下所示。
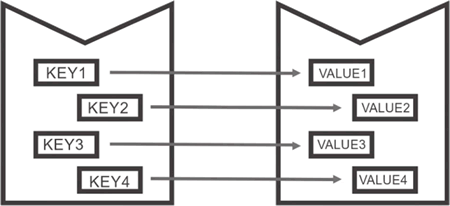
图 1 键值对
创建字典的语法如下:
下面创建字典:
当键重复时会产生错误,下面举一个例子:
下面定义一个字典,并将该字典赋值给 angel,通过访问键“语文”,输出字典对应的值:
下面将键“语文”改为“历史”:
我们可以使用 json.dumps() 函数将字典转换为 JSON 格式的字符串。例如:
如果想将 Unicode 字符串“\u82f1\u8bed”转换为中文,可使用 encode() 和 decode() 函数。例如:
字典是 Python 中唯一的一种映射类型,即元素之间是相互对应的。我们习惯将元素的索引称为键(key),键对应的元素称为值(value),键及其关联的值称为键值对。键值对示意图如下所示。
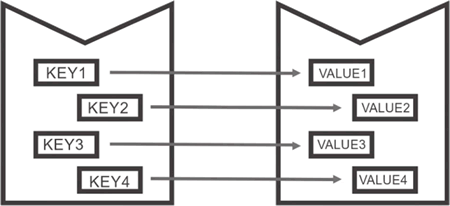
图 1 键值对
创建字典的语法如下:
dictname = {'key1':'value1', 'key2':'value2', ..., 'keyn':valuen}dictname 表示字典名,“keyn:valuen” 表示键值对。需要注意的是,同一字典中的键必须唯一,不能重复。
下面创建字典:
cloudy = {'语文': 99, '数学': 98, '英语': 95} print(cloudy)字典使用大括号表示,键使用单引号表示。运行结果为:
{'语文': 99, '数学': 98, '英语': 95}
当键重复时会产生错误,下面举一个例子:
cloudy = {'语文': 99, '语文': 98, '英语': 95} print(cloudy)键“语文”重复了两次,那么在输出字典时,只会显示一次值。运行结果为:
{'语文': 98, '英语': 95}
Python访问字典
访问字典是指通过访问指定的键,输出字典对应的值。下面定义一个字典,并将该字典赋值给 angel,通过访问键“语文”,输出字典对应的值:
cloudy = {'语文': 99, '数学': 98, '英语': 95} angel = dict(cloudy) print(angel['语文'])运行结果为:
99
下面将键“语文”改为“历史”:
cloudy = {'历史': 99, '数学': 98, '英语': 95} angel = dict(cloudy) print(angel['语文'])因为键“语文”不存在,所以运行程序会报错。
Python字典转换
字典可以转换为字符串,例如:cloudy = {'语文': 99, '数学': 98, '英语': 95} angel = str(cloudy) print(angel)此时字典已经成功转换为字符串,运行结果为:
{'语文': 99, '数学': 98, '英语': 95}
我们可以使用 json.dumps() 函数将字典转换为 JSON 格式的字符串。例如:
import json cloudy = {'yuwen': 99, 'shuxue': 98, '英语': 95} angel = json.dumps(cloudy) print(angel)运行结果为:
{"yuwen": 99, "shuxue": 98, "英语": 95}
使用 json.dumps() 函数后,键“英语”变为 Unicode 字符串“\u82f1\u8bed”。如果想将 Unicode 字符串“\u82f1\u8bed”转换为中文,可使用 encode() 和 decode() 函数。例如:
cloudy = r'\u82f1\u8bed' cloudycn = cloudy.encode('utf-8').decode('unicode_escape') print(cloudycn)运行结果为:
英语
Python删除字典
我们可使用 del() 函数删除字典,例如:cloudy = {'语文': 99, '数学': 98, '英语': 95} del(cloudy)